For the black industry of APP, we mentioned that some users will bypass the wind control strategy by changing IP. A more convenient way to change IP is to use proxy IP or VPN.
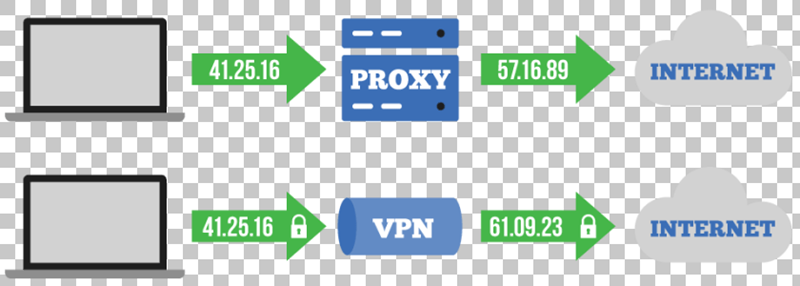
In testing APP security is required to make a judgment on whether to use the code and VPN. The following is a compilation of some codes for reference.
Android determine whether to use proxy IP
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
|
private boolean isWifiProxy(Context context) {
final boolean IS_ICS_OR_LATER = Build.VERSION.SDK_INT >= Build.VERSION_CODES.ICE_CREAM_SANDWICH;
String proxyAddress;
int proxyPort;
if (IS_ICS_OR_LATER) {
proxyAddress = System.getProperty("http.proxyHost");
String portStr = System.getProperty("http.proxyPort");
proxyPort = Integer.parseInt((portStr != null ? portStr : "-1"));
} else {
proxyAddress = android.net.Proxy.getHost(context);
proxyPort = android.net.Proxy.getPort(context);
}
return (!TextUtils.isEmpty(proxyAddress)) && (proxyPort != -1);
}
|
Android to determine whether to use VPN
1
2
3
4
5
6
7
|
boolean checkVPN(ConnectivityManager connMgr) {
//don't know why always returns null:
NetworkInfo networkInfo = connMgr.getNetworkInfo(ConnectivityManager.TYPE_VPN);
boolean isVpnConn = networkInfo == null ? false : networkInfo.isConnected();
return isVpnConn;
}
|
iOS to determine whether to use proxy IP
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
|
#import "CETCProxyStatus.h"
@implementation CETCProxyStatus
+ (BOOL)getProxyStatus {
NSDictionary *proxySettings = NSMakeCollectable([(NSDictionary *)CFNetworkCopySystemProxySettings() autorelease]);
NSArray *proxies = NSMakeCollectable([(NSArray *)CFNetworkCopyProxiesForURL((CFURLRef)[NSURL URLWithString:@"http://www.baidu.com"], (CFDictionaryRef)proxySettings) autorelease]);
NSDictionary *settings = [proxies objectAtIndex:0];
NSLog(@"host=%@", [settings objectForKey:(NSString *)kCFProxyHostNameKey]);
NSLog(@"port=%@", [settings objectForKey:(NSString *)kCFProxyPortNumberKey]);
NSLog(@"type=%@", [settings objectForKey:(NSString *)kCFProxyTypeKey]);
if ([[settings objectForKey:(NSString *)kCFProxyTypeKey] isEqualToString:@"kCFProxyTypeNone"]) {
//没有设置代理
return NO;
} else {
//设置代理了
return YES;
}
}
|
iOS determines if a VPN is being used
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
|
- (BOOL)isVPNOn
{
BOOL flag = NO;
NSString *version = [UIDevice currentDevice].systemVersion;
// need two ways to judge this.
if (version.doubleValue >= 9.0)
{
NSDictionary *dict = CFBridgingRelease(CFNetworkCopySystemProxySettings());
NSArray *keys = [dict[@"__SCOPED__"] allKeys];
for (NSString *key in keys) {
if ([key rangeOfString:@"tap"].location != NSNotFound ||
[key rangeOfString:@"tun"].location != NSNotFound ||
[key rangeOfString:@"ipsec"].location != NSNotFound ||
[key rangeOfString:@"ppp"].location != NSNotFound){
flag = YES;
break;
}
}
}
else
{
struct ifaddrs *interfaces = NULL;
struct ifaddrs *temp_addr = NULL;
int success = 0;
// retrieve the current interfaces - returns 0 on success
success = getifaddrs(&interfaces);
if (success == 0)
{
// Loop through linked list of interfaces
temp_addr = interfaces;
while (temp_addr != NULL)
{
NSString *string = [NSString stringWithFormat:@"%s" , temp_addr->ifa_name];
if ([string rangeOfString:@"tap"].location != NSNotFound ||
[string rangeOfString:@"tun"].location != NSNotFound ||
[string rangeOfString:@"ipsec"].location != NSNotFound ||
[string rangeOfString:@"ppp"].location != NSNotFound)
{
flag = YES;
break;
}
temp_addr = temp_addr->ifa_next;
}
}
// Free memory
freeifaddrs(interfaces);
}
return flag;
}
|