The bytes library in Go has two useful byte comparison functions: Compare, Equal
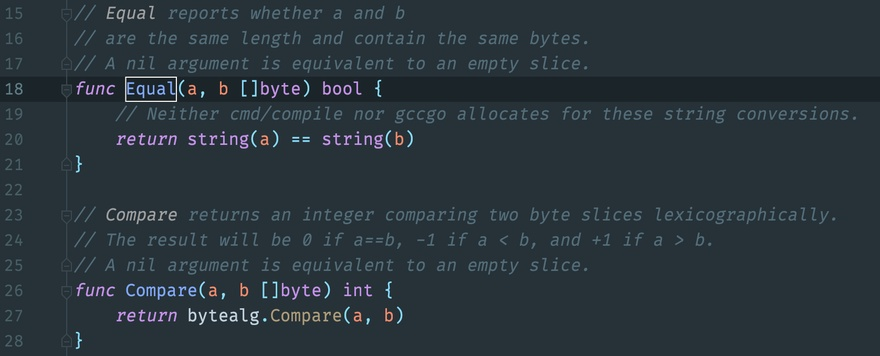
bytes.Compare
Compare is a comparison of the size of two [][]byte
s, returning the value
0
: a == b
-1
: a < b
+1
: a > b
bytes.Equal
Equal directly determines whether the two are equal, a simple task, and definitely faster than Compare
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
|
var bs1 = [][]byte{
[]byte("12a7.0.0.1:109e2"),
[]byte("127.ss.0.aw:1092"),
[]byte("12a.r.0.1:1092cd"),
[]byte("127.0.0.1:1092"),
[]byte("127.s.0.aw:1092"),
[]byte("12a.r.0.1:1092c"),
[]byte("127.0.0.1:1092"),
[]byte("127.s.0.aw:1092"),
[]byte("12a.r.0.1:1092c"),
}
var bs2 = [][]byte{
[]byte("12a7.0.0.1:109e2"),
[]byte("127.ss.0.aw:1092"),
[]byte("12a.r.0.1:1092cd"),
[]byte("127.0.0.1:1092"),
[]byte("127.s.0.aw:1092"),
[]byte("12a.r.0.1:1092c"),
[]byte("12a7.0.0.1:109e2"),
[]byte("127.ss.0.aw:1092"),
[]byte("12a.r.0.1:1092cd"),
}
func Benchmark_bytes_compare(b *testing.B) {
b.ResetTimer()
defer b.StopTimer()
for i := 0; i < b.N; i++ {
for j, s := range bs1 {
if bytes.Compare(s, bs2[j]) != 0 {
// do nothing
}
}
}
}
func Benchmark_bytes_equal(b *testing.B) {
b.ResetTimer()
defer b.StopTimer()
for i := 0; i < b.N; i++ {
for j, s := range bs1 {
if !bytes.Equal(s, bs2[j]) {
// do nothing
}
}
}
|
Run test
1
|
go test -v compare_test.go -bench "Benchmark" -benchmem
|
Results.
1
2
3
4
5
6
7
|
goos: darwin
goarch: amd64
cpu: Intel(R) Core(TM) i7-4870HQ CPU @ 2.50GHz
Benchmark_bytes_compare
Benchmark_bytes_compare-8 27013251 43.00 ns/op 0 B/op 0 allocs/op
Benchmark_bytes_equal
Benchmark_bytes_equal-8 36742129 29.03 ns/op 0 B/op 0 allocs/op
|
As expected, the results were much faster, so let’s use equal where we can.