The family bought tiny blocks for the kids to play with, and since the instructions that came with them only had a few pictures, they were finished after a few spellings, so I planned to use Python to make a pixel painting picture tool.
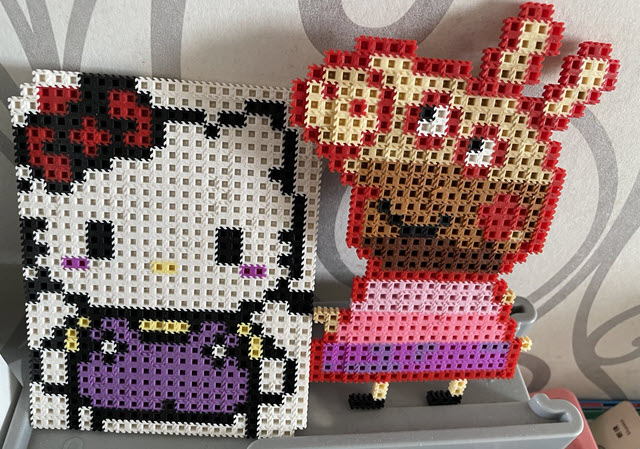
The overall logic is very simple.
- Read the image using the Pillow package
- Split the image into a square block of pixels
- Get the color that appears most in the pixel block and use it as the color of the pixel block.
- Re-splicing the image.
The specific code is as follows.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
|
from PIL import Image
import collections
pic = Image.open("image.png").convert("RGB")
width, height = pic.size
block = 10 # Set block size
board = Image.new("RGB", (width // block * block, height // block * block))
for y in range(0, height, block):
for x in range(0, width, block):
temp = pic.crop((x, y, x + block, y + block))
temp_list = list(temp.getdata())
max_color = collections.Counter(temp_list).most_common()[0][0]
# temp_past = Image.new("RGB", temp.size, max_color) Borderless
temp_past = Image.new("RGB", (temp.size[0] - 1, temp.size[1] - 1), max_color)
board.paste(temp_past, (x, y))
board.show()
|
The specific effect is as follows.
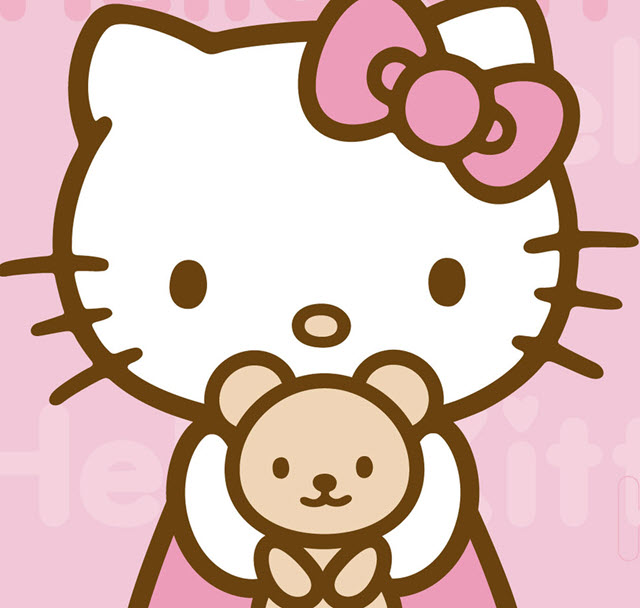
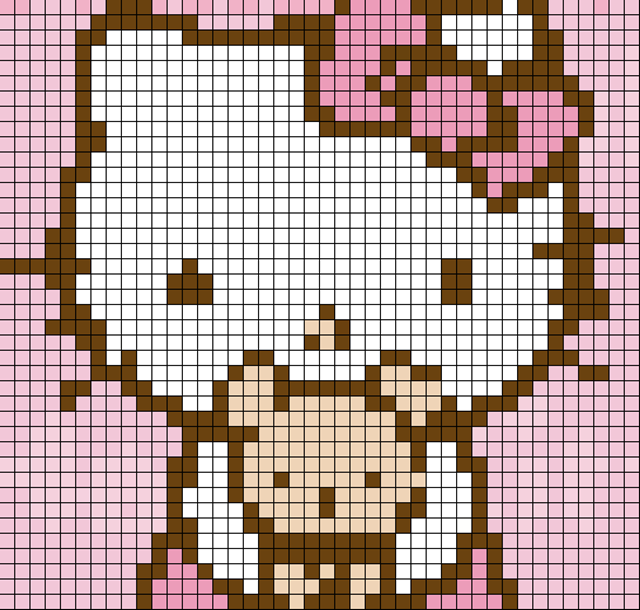
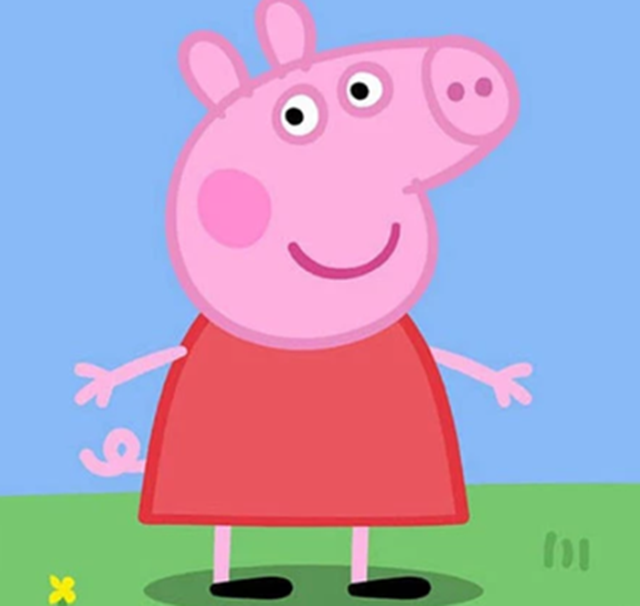
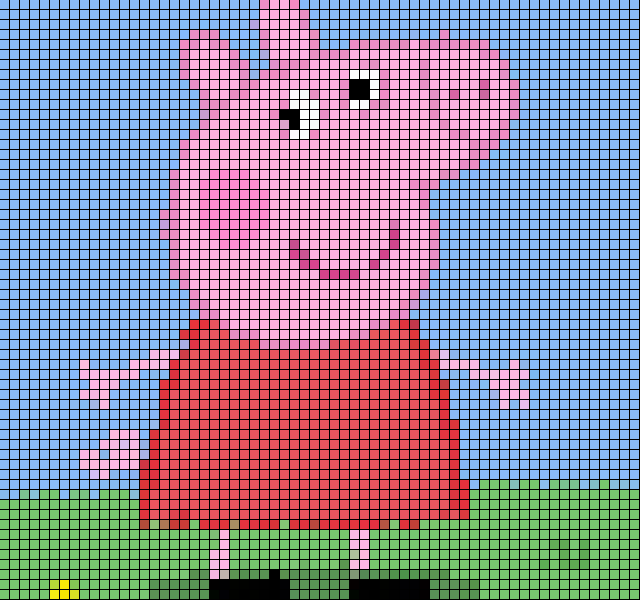