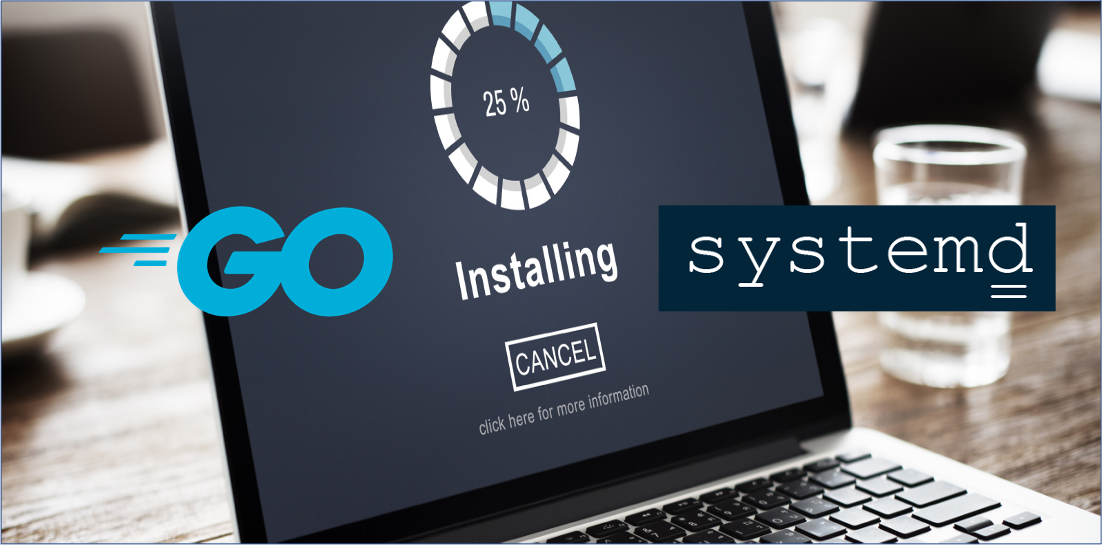
In the article “Getting reviewdog to support gitlab-push-commit”, gitlab-runner (a Go language developed application) installs itself as a system service via its own provided install command as a system service (as in the following steps).
1
2
3
4
5
6
|
# Create a GitLab CI user
sudo useradd --comment 'GitLab Runner' --create-home gitlab-runner --shell /bin/bash
# Install and run as service
sudo gitlab-runner install --user=gitlab-runner --working-directory=/home/gitlab-runner
sudo gitlab-runner start
|
In the mainstream new version of linux (other os or linux on the old version of the daemon service manager such as sysvinit, upstart, etc., we do not care for now), the system service is the daemon process managed by systemd.
After the linux host is powered on, the os kernel is loaded and started, and after the os kernel is initialized, the first program started by the kernel is the init program, whose PID (process ID) is 1, and it is the “ancestor” of all processes in the system. systemd is the mainstream new version of the init program in linux. systemd is the init program in newer versions of mainstream Linux, which is responsible for pulling up all the programs installed as system services after the host starts.
These services pulled up by systemd run as daemon processes, so what are daemons? The book Advanced Programming in the UNIX Environment (3rd Edition) defines it as follows.
Daemons are processes that live for a long time. They are often started when the system is bootstrapped and terminate only when the system is shut down. Because they don’t have a controlling terminal, we say that they run in the background. UNIX systems have numerous daemons that perform day-to-day activities.
The book also provides a standard procedure (coding rules) for a user-level application to turn itself into a daemon, and gives a C example.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
|
#include "apue.h"
#include <syslog.h>
#include <fcntl.h>
#include <sys/resource.h>
void
daemonize(const char *cmd)
{
int i, fd0, fd1, fd2;
pid_t pid;
struct rlimit rl;
struct sigaction sa;
/*
* Clear file creation mask.
*/
umask(0);
/*
* Get maximum number of file descriptors.
*/
if (getrlimit(RLIMIT_NOFILE, &rl) < 0)
err_quit("%s: can't get file limit", cmd);
/*
* Become a session leader to lose controlling TTY.
*/
if ((pid = fork()) < 0)
err_quit("%s: can't fork", cmd);
else if (pid != 0) /* parent */
exit(0);
setsid();
/*
* Ensure future opens won't allocate controlling TTYs.
*/
sa.sa_handler = SIG_IGN;
sigemptyset(&sa.sa_mask);
sa.sa_flags = 0;
if (sigaction(SIGHUP, &sa, NULL) < 0)
err_quit("%s: can't ignore SIGHUP", cmd);
if ((pid = fork()) < 0)
err_quit("%s: can't fork", cmd);
else if (pid != 0) /* parent */
exit(0);
/*
* Change the current working directory to the root so
* we won't prevent file systems from being unmounted.
*/
if (chdir("/") < 0)
err_quit("%s: can't change directory to /", cmd);
/*
* Close all open file descriptors.
*/
if (rl.rlim_max == RLIM_INFINITY)
rl.rlim_max = 1024;
for (i = 0; i < rl.rlim_max; i++)
close(i);
/*
* Attach file descriptors 0, 1, and 2 to /dev/null.
*/
fd0 = open("/dev/null", O_RDWR);
fd1 = dup(0);
fd2 = dup(0);
/*
* Initialize the log file.
*/
openlog(cmd, LOG_CONS, LOG_DAEMON);
if (fd0 != 0 || fd1 != 1 || fd2 != 2) {
syslog(LOG_ERR, "unexpected file descriptors %d %d %d",
fd0, fd1, fd2);
exit(1);
}
}
|
So, is it possible for a Go application to convert itself to a daemon by following the conversion steps above? Unfortunately, the Go team says it’s very difficult to do so. there are many third-party solutions available in the Go community, such as third-party implementations like go-daemon, but I haven’t verified them and can’t guarantee that they are completely OK.
The Go team recommends an init system like systemd for daemon conversion of Go programs. gitlab-runner installs itself as a system service and is managed by systemd.
Off-topic: Actually, since the container technology (e.g.: docker), the need for daemon service seems to have decreased. Because with the -d
option to run the container, the application itself runs in the background, and with the -restart=always/on-failure
option, the container engine (e.g. docker engine) will manage the service for us and restart the service after it goes down.
So, how do we install our application as a systemd service like gitlab-runner? Let’s continue down the list.
Note: Here you are only installing the Go application as a systemd service, not converting it to a daemon. Installing it as a systemd service itself is possible and safe.
Looking at the gitlab-runner source code, you will find that gitlab-runner will install itself as a system service all rely on github.com/kardianos/service this Go package, this package is the Go standard library database package one of the maintainers Daniel Theophanes open source, operating system Service package, the package shields the os layer differences, providing developers with a relatively simple Service operation interface, including the following control actions.
1
2
|
// github.com/kardianos/service/blob/master/service.go
var ControlAction = [5]string{"start", "stop", "restart", "install", "uninstall"}
|
Well, let’s use an example myapp to introduce how to use the kardianos/service package to give your Go application the ability to install itself as a system service.
myapp is an http server that serves on a port and returns a “Welcome” response when a request is received.
1
2
3
4
5
6
7
8
9
10
11
12
|
// https://github.com/bigwhite/experiments/blob/master/system-service/main.go
func run(config string) error {
... ...
http.HandleFunc("/", func(w http.ResponseWriter, r *http.Request) {
fmt.Printf("[%s]: receive a request from: %s\n", c.Server.Addr, r.RemoteAddr)
w.Write([]byte("Welcome"))
})
fmt.Printf("listen on %s\n", c.Server.Addr)
return http.ListenAndServe(c.Server.Addr, nil)
}
|
Now we want to add some capabilities to myapp so that it supports installing itself as a systemd service and can start, stop and uninstall the systemd service with a subcommand.
We start by adding parsing capabilities to the program for subcommand and its arguments via the os package and the flag package. We do not use a third-party command-line parameter parsing package, but just the flag package from the standard library. Since myapp supports subcommand, we need to apply a separate FlagSet instance for each subcommand with command line arguments, such as installCommand and runCommand in the following code. The command line arguments of each subcommand are also bound to the corresponding FlagSet instance of the respective subcommand, as shown in the init function body of the following code.
In addition, since we are using a subcommand, the default flag.Usage no longer meets our requirements, so we need to implement our own usage function and assign it to flag.Usage.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
|
// https://github.com/bigwhite/experiments/blob/master/system-service/main.go
var (
installCommand = flag.NewFlagSet("install", flag.ExitOnError)
runCommand = flag.NewFlagSet("run", flag.ExitOnError)
user string
workingdir string
config string
)
const (
defaultConfig = "/etc/myapp/config.ini"
)
func usage() {
s := `
USAGE:
myapp command [command options]
COMMANDS:
install install service
uninstall uninstall service
start start service
stop stop service
run run service
OPTIONS:
-config string
config file of the service (default "/etc/myapp/config.ini")
-user string
user account to run the service
-workingdir string
working directory of the service`
fmt.Println(s)
}
func init() {
installCommand.StringVar(&user, "user", "", "user account to run the service")
installCommand.StringVar(&workingdir, "workingdir", "", "working directory of the service")
installCommand.StringVar(&config, "config", "/etc/myapp/config.ini", "config file of the service")
runCommand.StringVar(&config, "config", defaultConfig, "config file of the service")
flag.Usage = usage
}
func main() {
var err error
n := len(os.Args)
if n <= 1 {
fmt.Printf("invalid args\n")
flag.Usage()
return
}
subCmd := os.Args[1] // the second arg
// get Config
c, err := getServiceConfig(subCmd)
if err != nil {
fmt.Printf("get service config error: %s\n", err)
return
}
... ...
}
|
After all this is done, we get the meta-configuration information of this service that will be installed as systemd service in the getServiceConfig function.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
|
// https://github.com/bigwhite/experiments/blob/master/system-service/config.go
func getServiceConfig(subCmd string) (*service.Config, error) {
c := service.Config{
Name: "myApp",
DisplayName: "Go Daemon Service Demo",
Description: "This is a Go daemon service demo",
Executable: "/usr/local/bin/myapp",
Dependencies: []string{"After=network.target syslog.target"},
WorkingDirectory: "",
Option: service.KeyValue{
"Restart": "always", // Restart=always
},
}
switch subCmd {
case "install":
installCommand.Parse(os.Args[2:])
if user == "" {
fmt.Printf("error: user should be provided when install service\n")
return nil, errors.New("invalid user")
}
if workingdir == "" {
fmt.Printf("error: workingdir should be provided when install service\n")
return nil, errors.New("invalid workingdir")
}
c.UserName = user
c.WorkingDirectory = workingdir
// arguments
// ExecStart=/usr/local/bin/myapp "run" "-config" "/etc/myapp/config.ini"
c.Arguments = append(c.Arguments, "run", "-config", config)
case "run":
runCommand.Parse(os.Args[2:]) // parse config
}
return &c, nil
}
|
Note here the Option and Arguments in service.Config. The former is used to place arbitrary key-value pairs in the systemd service unit configuration file (e.g. Restart=always here), while the Arguments will be composed as the value of the ExecStart key, which will will be passed in when the service is started.
Next, we use the service package to create an instance of the operation service (srv) based on the loaded Config, and then pass it into the runServiceControl along with the subCommand to achieve control of the systemd service (as in the code below).
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
|
// https://github.com/bigwhite/experiments/blob/master/system-service/main.go
func main() {
// ... ...
c, err := getServiceConfig(subCmd)
if err != nil {
fmt.Printf("get service config error: %s\n", err)
return
}
prg := &NullService{}
srv, err := service.New(prg, c)
if err != nil {
fmt.Printf("new service error: %s\n", err)
return
}
err = runServiceControl(srv, subCmd)
if err != nil {
fmt.Printf("%s operation error: %s\n", subCmd, err)
return
}
fmt.Printf("%s operation ok\n", subCmd)
return
}
func runServiceControl(srv service.Service, subCmd string) error {
switch subCmd {
case "run":
return run(config)
default:
return service.Control(srv, subCmd)
}
}
|
Okay, the code is done! Now let’s verify the capabilities of myapp.
Let’s start by completing the compilation and installation of the binary.
1
2
3
4
5
6
7
8
|
$make
go build -o myapp main.go config.go
$sudo make install
cp ./myapp /usr/local/bin
$sudo make install-cfg
mkdir -p /etc/myapp
cp ./config.ini /etc/myapp
|
Next, let’s install myapp as a service of systemd.
1
2
3
4
5
6
7
|
$sudo ./myapp install -user tonybai -workingdir /home/tonybai
install operation ok
$sudo systemctl status myApp
● myApp.service - This is a Go daemon service demo
Loaded: loaded (/etc/systemd/system/myApp.service; enabled; vendor preset: enabled)
Active: inactive (dead)
|
We see that after installation, myApp has become myApp.service and is in the inactive state, and its systemd unit file /etc/systemd/system/myApp.service
has the following contents.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
|
$sudo cat /etc/systemd/system/myApp.service
[Unit]
Description=This is a Go daemon service demo
ConditionFileIsExecutable=/usr/local/bin/myapp
After=network.target syslog.target
[Service]
StartLimitInterval=5
StartLimitBurst=10
ExecStart=/usr/local/bin/myapp "run" "-config" "/etc/myapp/config.ini"
WorkingDirectory=/home/tonybai
User=tonybai
Restart=always
RestartSec=120
EnvironmentFile=-/etc/sysconfig/myApp
[Install]
WantedBy=multi-user.target
|
Next, let’s start the service.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
|
$sudo ./myapp start
start operation ok
$sudo systemctl status myApp
● myApp.service - This is a Go daemon service demo
Loaded: loaded (/etc/systemd/system/myApp.service; enabled; vendor preset: enabled)
Active: active (running) since Fri 2022-09-09 23:30:01 CST; 5s ago
Main PID: 623859 (myapp)
Tasks: 6 (limit: 12651)
Memory: 1.3M
CGroup: /system.slice/myApp.service
└─623859 /usr/local/bin/myapp run -config /etc/myapp/config.ini
Sep 09 23:30:01 tonybai systemd[1]: Started This is a Go daemon service demo.
Sep 09 23:30:01 tonybai myapp[623859]: listen on :65432
|
We see that the myApp service started successfully and is listening on the port 65432!
We use curl to send a request to this port.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
|
$curl localhost:65432
Welcome
$sudo systemctl status myApp
● myApp.service - This is a Go daemon service demo
Loaded: loaded (/etc/systemd/system/myApp.service; enabled; vendor preset: enabled)
Active: active (running) since Fri 2022-09-09 23:30:01 CST; 1min 27s ago
Main PID: 623859 (myapp)
Tasks: 6 (limit: 12651)
Memory: 1.4M
CGroup: /system.slice/myApp.service
└─623859 /usr/local/bin/myapp run -config /etc/myapp/config.ini
Sep 09 23:30:01 tonybai systemd[1]: Started This is a Go daemon service demo.
Sep 09 23:30:01 tonybai myapp[623859]: listen on :65432
Sep 09 23:31:24 tonybai myapp[623859]: [:65432]: receive a request from: 127.0.0.1:10348
|
We see that the myApp service is running fine and returning the expected response.
Now we stop the service using the stop subcommand.
1
2
3
4
5
6
7
8
9
10
11
12
13
|
$sudo systemctl status myApp
● myApp.service - This is a Go daemon service demo
Loaded: loaded (/etc/systemd/system/myApp.service; enabled; vendor preset: enabled)
Active: inactive (dead) since Fri 2022-09-09 23:33:03 CST; 3s ago
Process: 623859 ExecStart=/usr/local/bin/myapp run -config /etc/myapp/config.ini (code=killed, signal=TERM)
Main PID: 623859 (code=killed, signal=TERM)
Sep 09 23:30:01 tonybai systemd[1]: Started This is a Go daemon service demo.
Sep 09 23:30:01 tonybai myapp[623859]: listen on :65432
Sep 09 23:31:24 tonybai myapp[623859]: [:65432]: receive a request from: 127.0.0.1:10348
Sep 09 23:33:03 tonybai systemd[1]: Stopping This is a Go daemon service demo...
Sep 09 23:33:03 tonybai systemd[1]: myApp.service: Succeeded.
Sep 09 23:33:03 tonybai systemd[1]: Stopped This is a Go daemon service demo.
|
Modify the configuration /etc/myapp/config.ini (change the listening port from 65432 to 65431) and then restart the service.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
|
$sudo cat /etc/myapp/config.ini
[server]
addr=":65431"
$sudo ./myapp start
start operation ok
$sudo systemctl status myApp
● myApp.service - This is a Go daemon service demo
Loaded: loaded (/etc/systemd/system/myApp.service; enabled; vendor preset: enabled)
Active: active (running) since Fri 2022-09-09 23:34:38 CST; 3s ago
Main PID: 624046 (myapp)
Tasks: 6 (limit: 12651)
Memory: 1.4M
CGroup: /system.slice/myApp.service
└─624046 /usr/local/bin/myapp run -config /etc/myapp/config.ini
Sep 09 23:34:38 tonybai systemd[1]: Started This is a Go daemon service demo.
Sep 09 23:34:38 tonybai myapp[624046]: listen on :65431
|
From the systemd status log we see that the myApp service started successfully and changed to listen on port 65431, let’s access that port.
1
2
3
4
5
|
$curl localhost:65431
Welcome
$curl localhost:65432
curl: (7) Failed to connect to localhost port 65432: Connection refused
|
As you can see from the above results, our configuration update and reboot were successful!
We can also uninstall the service from systemd using the uninstall function of myapp.
1
2
3
4
|
$sudo ./myapp uninstall
uninstall operation ok
$sudo systemctl status myApp
Unit myApp.service could not be found.
|
Well, here we see: the goal proposed at the beginning of the article to add the ability for Go applications to install themselves as systemd services has been successfully achieved.
The code covered in this article can be downloaded at here.
Reference
https://tonybai.com/2022/09/12/how-to-install-a-go-app-as-a-system-service-like-gitlab-runner/